* feat: Add support for emitting literal C This commit adds a new Obj type, C, as well as a command for emitting C literals (which are represented by this object). This is necessary for circumstances in which Carp's handling of templates and emission orders prevents interop with C. For example, the c11 macro static_assert must only take a string literal in its second position. However, Carp's memory management will typically assign a string literal (in Carp) to an anonymous variable, which makes them impossible to use with static_assert. The new emit-c command will support this use case: ``` (static-assert 0 (Unsafe.emit-c "\"message!\"")) ``` The literal string "message!" will be emitted in the compiler's C output in the position corresponding to the macro call. We also add a special type for c literals, CTy, to prevent conflating them with Strings. This helps maintainers define clear boundaries and express what interop code requires the use of literal C. Likewise, we need to emit `c_code` to represent this type in C. This wouldn't be necessary except that Carp sometimes auto-generates functions that refer to Carp types in their C equivalents, so we need this for completeness. It is typed as the void pointer. N.B. That the command is not yet exposed in Carp in this commit. Thanks to @TimDeve for recommending the name emit-c! * feat: Add preproc command and add emit-c to Unsafe module This commit adds the preproc command, which enables users to emit arbitrary C in the compilers emitted C output. The C emitted by calls to preproc will be appended to the output after include directives but prior to any other emissions. One can use it to call preprocessor directives in C, define helper functions etc. preproc takes a C type value as an argument and must be used in conjunction with emit-c. Both functions are added to the Unsafe module to signal their dangerousness. One can use `register` in combination with preproc to define code entirely in Carp and obviate the need of additional header files. For example: ``` (Unsafe.preproc (Unsafe.emit-c "#define FOO 0")) (Unsafe.preproc (Unsafe.emit-c "void foo() { printf(\"%d\\n\", 1); }")) (register FOO Int) (register foo (Fn [] ())) (defn main [] (do (foo) (IO.println &(fmt "%d" FOO)))) ``` The prior example emits C that defines a FOO macro and foo function, which are both referenced in the main function emitted by Carps normal processing. Here's what the output looks like: ``` // .. several other includes emitted by the compiler... void foo() { printf("%d\n", 1); } //Types: // Depth 3 typedef struct { union { struct { Long member0; } Just; // Nothing char __dummy; } u; char _tag; } Maybe__Long; ``` The C passed to preproc calls is emitted prior to Carps other emissions, ensuring the user has access to these definitions before any Carp code is called. * docs: Add documentation on emit-c and preproc * feat: add macros for emitting C compiler directives This suite of macros uses the `Unsafe.emit-c` and `Unsafe.preproc` functions to provide macros for emitting common C compiler directives, such as #ifdef, #define, #pragma and others. |
||
---|---|---|
.github | ||
app | ||
bench | ||
core | ||
docs | ||
examples | ||
headerparse | ||
resources | ||
scripts | ||
src | ||
test | ||
.build.yml | ||
.clang-format | ||
.dir-locals.el | ||
.gitignore | ||
.travis.yml | ||
CarpHask.cabal | ||
default.nix | ||
LICENSE | ||
LUA_LICENSE | ||
README.md | ||
Setup.hs | ||
stack.yaml |
Carp
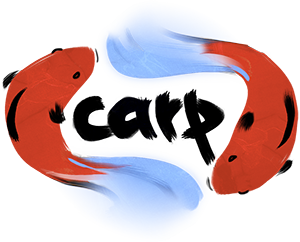
WARNING! This is a research project and a lot of information here might become outdated and misleading without any explanation. Don't use it for anything important just yet!
Version 0.5 of the language is out!
About
Carp is a programming language designed to work well for interactive and performance sensitive use cases like games, sound synthesis and visualizations.
The key features of Carp are the following:
- Automatic and deterministic memory management (no garbage collector or VM)
- Inferred static types for great speed and reliability
- Ownership tracking enables a functional programming style while still using mutation of cache-friendly data structures under the hood
- No hidden performance penalties – allocation and copying are explicit
- Straightforward integration with existing C code
- Lisp macros, compile time scripting and a helpful REPL
Learn more
- The Compiler Manual - how to install and use the compiler
- Carp Language Guide - syntax and semantics of the language
- Core Docs - documentation for our standard library
A Very Small Example
(load-and-use SDL)
(defn tick [state]
(+ state 10))
(defn draw [app rend state]
(bg rend &(rgb (/ @state 2) (/ @state 3) (/ @state 4))))
(defn main []
(let [app (SDLApp.create "The Minimalistic Color Generator" 400 300)
state 0]
(SDLApp.run-with-callbacks &app SDLApp.quit-on-esc tick draw state)))
For instructions on how to run Carp code, see this document.
For more examples, check out the examples directory.
Maintainers
Contributing
Thanks to all the awesome people who have contributed to Carp over the years!
We are always looking for more help – check out the contributing guide to get started.
License
Copyright 2016 - 2020 Erik Svedäng
Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
The regular expression implementation as found in src/carp_regex.h are Copyright (C) 1994-2017 Lua.org, PUC-Rio under the terms of the MIT license. Details can be found in the License file LUA_LICENSE.