Simplified layout algorithm by removing `content_origin`, and instead treating `(0.0, 0.0)` as origin point in every layout object. This change allows overflowing containers that are within auto-layout. The parent element will no longer be moved within the grid cell when its children overflow it.
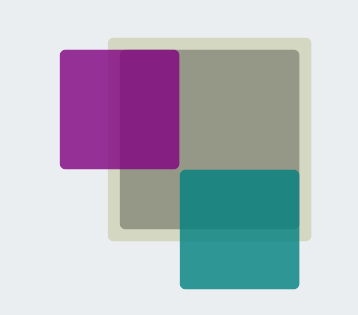
# Important Notes
When implementing this change, I have found that when object's size was modified without ever touching its position, that change was not being picked up in the "modified children" list, and `on_updated` was never triggered. Because some sprites now are bottom-left aligned, that is now a common case and was reproducible on the auto-layout example scene. I ended up fixing it by introducing another dirty flag for `computed_size` changes. Right now that flag is applied very broadly (on each layout update), but in the future we might make it more precise by actually checking if the size was changed in the process.
I believe that this might also be a fix for #5095, as I cannot reproduce it anymore with those changes.
Implements https://www.pivotaltracker.com/n/projects/2539304/stories/184023380
Dropdown component. Planned to be used in nodes as a single and multiple selection widget, both for static and dynamically loaded values. Initial support is focused on static data, with limited support for dynamic sources. Notably, loading states are not supported yet. Full support for that is planned to be added later with widget lazy-loading.
- Supports single and multiple selections.
- Dedicated API for providing a static list of all entries.
- Range-based query API for dynamically loading data as it is scrolled (only basic support - will need more work for proper async lazy-loading).
- Internal entry cache and query batching to avoid querying data one by one (the batching for now is very basic, will have to be improved for proper lazy-loading).
- Automatic dropdown width adjustment based on the entry label lengths, up to a set max allowed value.
- Open and close animation.
- Keyboard support for focusing and selecting entries.
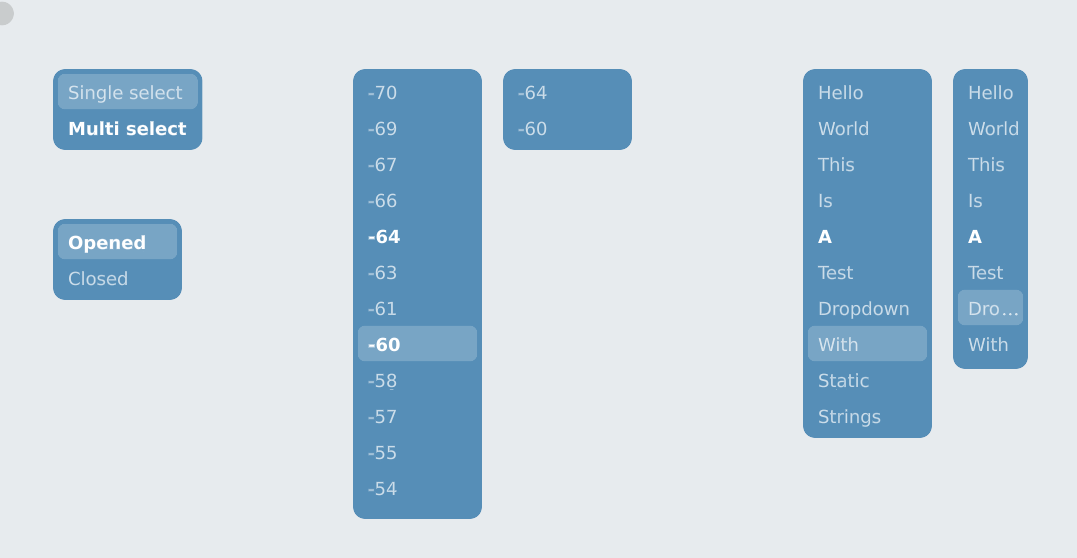
# Important Notes
Implementing the dropdown on top of grid-view have uncovered some assumptions around grid-view layers. It was assumed to always be a part of the component browser. Removing that assumption required a mechanism for propagating camera update information through layer tree. This is now implemented using a `camera_parent` layer field. Ideally each layer should simply have at most a single parent, and camera inheritance would follow that. That refactor turned out to be quite involved, so right now the simpler temporary solution is introduced in order to not delay this PR further.
When a GridView is navigated using the keyboard, scroll it to display the newly selected entry.
https://www.pivotaltracker.com/story/show/182593635
#### Visuals
See below for a video demonstrating automatic scrolling of the GridView when arrow keys are pressed on the keyboard. The first video below shows the default scrolling behavior in a GridView without headers.
Note:
- When the Grid View is scrolled, the mouse hover highlight moves away from the mouse position. This is not a new regression, the behavior is the same in the `develop` branch (e.g. when scrolling using the mouse).
https://user-images.githubusercontent.com/273837/190183984-91f7808c-3606-43f8-bcda-ac4d5f84e00f.mov
The video below shows the behavior in a GridView with headers when the GridView is first scrolled to its top-left corner. The following guidance from the Design Doc is enabled and showcased:
> Users can change the selected component by pressing the arrow keys. The Focus does not move up if it does not have to (in most cases, the focus is located in the second row from the bottom). Instead, the component list scrolls down if there are enough entries.
https://user-images.githubusercontent.com/273837/189151546-e50aaf22-6f4d-41cb-809f-60038305745f.mov
The next video shows the behavior in the same GridView as in the previous example when the GridView is first scrolled away from any of its boundary entries. Notably, scrolling happens only when the selection is moved using the keyboard arrow keys, not when changing the selection using the mouse. This behavior is based on a discussion with @wdanilo on Discord.
https://user-images.githubusercontent.com/273837/189151974-d992be93-f61f-4e9f-9f4c-dfe260bbec5b.mov
This PR adds a new variant of selection, where the mouse-hovered entry is highlighted and may be selected by clicking.
In the video below, we have three grid views with slightly different settings:
* In the left-top corner, both hover and selection highlight is just a shape under the label. Such a grid view does not require additional layers (when compared to non-selectable grid view).
* In the left-bottom corner the hover is normal shape, but selection is a _masked layer_ which allows us to have different text color. This setting requires three more layers to render.
* In the right-top corner, both hover and selection are displayed in the masked layer, creating 6 additional layers.
https://user-images.githubusercontent.com/3919101/181514178-f243bfeb-f2dd-4507-adc3-5344ae0579b7.mp4
**Note**: This PR also contains content of previous Grid View PR. We decided to discard the previous, because this one did some refactoring of old one, and it's not a big addition.
Added a scrollable::GridView component, which just embeds the GridView in ScrollArea. Also, re-worked the idea of text layers.
https://user-images.githubusercontent.com/3919101/179020359-512ee127-c333-4f86-bff5-f1cb4154e03c.mp4
This PR contains all work for finishing integration of first Component List Panel in the IDE:
* It adds a stub for the whole Component Browser View. The documentation panel is re-used from the old searcher.
* It has the presenter implementation, integrating the view with Hierarchical Component List from the controller.
* It extends the View API, so the integration is possible, making use of Component Group Set wrapper.
* The selection integration was also merged into this PR, because it depended on the API extension mentioned above. However, we should avoid such practice in the future.
https://user-images.githubusercontent.com/3919101/177816427-8c4285b4-8941-4048-a400-52f4acf77a9f.mp4
# Important Notes
There are some known issues, to-be-fixed in the future.
* The performance is bad. It should be improved with new text::Area, and the decent one shall come with [GridView inside component browser](https://www.pivotaltracker.com/story/show/182561072)
* There is no keyboard navigation. It should also be delivered with [GridView](https://www.pivotaltracker.com/story/show/182561072).
* The Favorites section is not [filtered out by node source type](https://www.pivotaltracker.com/story/show/182661634).
[ci no changelog needed]
[Task link](https://www.pivotaltracker.com/story/show/181725003)
This PR implements a fully visible component group header while scrolling the group (using the ScrollArea).
The header moves in sync with scrolling movements (using new `set_header_pos` FRP input), so it looks like the component group is scrolled. ScrollArea masks the "scrolled" entries above the header. This design allows a fully visible header even though our renderer doesn't support nested layers masking yet.
The screencast:
https://user-images.githubusercontent.com/6566674/168320360-2c2017b2-0ef5-42ce-9c79-82b9641c1d73.mp4
The most recent one, with the updated demo scene from develop:
https://user-images.githubusercontent.com/6566674/168555268-8552c4b0-f887-4388-89a1-e65ddf668be6.mp4
# Important Notes
- I fixed the API of the list view so now it supports non-hardcoded scene layers (previously it did not). I also believe it was implemented incorrectly.
- I've found a [pretty weird bug](https://www.pivotaltracker.com/story/show/182193824): the component group inside the ScrollArea is invisible unless I add some arbitrary shape to the scroll area content. I use a `transparent_circle` for this purpose in the demo scene. The bug is probably related to masking the sublayers, though I wasn't able to reproduce it properly on a simpler example.
- The selection box is removed from the demo scene as agreed with @farmaazon . The correct implementation has proven to be much harder than I expected, and we will implement another approach in a separate PR.
- I also modified the `shadow::Parameters` so that it uses `Var`s instead of plain values.
[ci no changelog needed]
This is fixed copy of already reviewed #3384
[Task link](https://www.pivotaltracker.com/story/show/181413200)
This PR implements content clipping for the ScrollArea component.
List of changes:
- Implemented `InstanceWithAttachedLayer` abstraction that allows creating additional sublayers for our components. In the future, this abstraction can be used for text rendering as well (right now text rendering requires additional hardcoded layers).
- Fixed `complex-shape-system` demo scene by removing `node_searcher_mask` layer.
- Fixed `SublayersModel::remove` - it was not clearing the `layer_placement` hashmap.
- Implemented disabling the wheel scrolling in `Navigator`, and refactored it to reduce the number of functions arguments by introducing a `NavigatorSettings` struct.
Video (`scroll_area` demo):
https://user-images.githubusercontent.com/6566674/164506455-e177a7a7-9f1c-4f50-888f-112423cebbe4.mp4
# Important Notes
- `InstanceWithAttachedLayer` is implemented in such a way that it allows an extension in the future - namely to use it to simplify text rendering. The implementation might be simplified though.
[ci no changelog needed]
[Task link](https://www.pivotaltracker.com/story/show/181413200)
This PR implements content clipping for the ScrollArea component.
List of changes:
- Implemented `InstanceWithAttachedLayer` abstraction that allows creating additional sublayers for our components. In the future, this abstraction can be used for text rendering as well (right now text rendering requires additional hardcoded layers).
- Fixed `complex-shape-system` demo scene by fixing `node_searcher_mask` layer.
- Fixed `SublayersModel::remove` - it was not clearing the `layer_placement` hashmap.
- Implemented disabling the wheel scrolling in `Navigator`, and refactored it to reduce the number of functions arguments by introducing a `NavigatorSettings` struct.
Video (`scroll_area` demo):
https://user-images.githubusercontent.com/6566674/164506455-e177a7a7-9f1c-4f50-888f-112423cebbe4.mp4
# Important Notes
- `InstanceWithAttachedLayer` is implemented in such a way that it allows an extension in the future - namely to use it to simplify text rendering. The implementation might be simplified though.